useClipboard
클립보드
와 상호작용하는 함수들과 저장된 데이터를 포함한 객체를 반환합니다.
클립보드에 복사된 데이터는 copiedData
상태에 저장됩니다. copyText
와 copyImage
는 성공 여부(Boolean)
를 반환합니다.
copyText
는 주어진 텍스트를 클립보드에 복사하는 함수입니다.copyImage
는 주어진 이미지 URL을 클립보드에 복사하는 함수입니다.
클립보드에서 읽어온 데이터는 readData
상태에 저장됩니다. readText
와 readContents
는 성공 여부(Boolean)
를 반환합니다.
readText
는 클립보드에 저장된 텍스트 데이터를 읽어오는 함수입니다.readContents
는 클립보드에 저장된 텍스트를 포함한 html, 이미지 등 다양한 유형의 컨텐츠를 읽어오는 함수입니다.
Code
Interface
typescript
function useClipboard(): {
copiedData: string | Blob | null;
readData: string | ClipboardItems | null;
readText: () => Promise<boolean>;
readContents: () => Promise<boolean>;
copyText: (value: string) => Promise<boolean>;
copyImage: (src: string, options?: { toText: boolean }) => Promise<boolean>;
};
Usage
typescript
import { useClipboard } from '@modern-kit/react';
import img from '../../assets/img.png';
const Example = () => {
const { copiedData, readData, copyText, copyImage, readContents, readText } = useClipboard();
const [text, setText] = useState('');
const handleCopyText = async () => {
const isSuccess = await copyText(text);
alert(`isSuccess: ${isSuccess}, 클립보드에 복사됐습니다. 브라우저 콘솔을 확인해주세요.`);
};
const handleCopyImage = async () => {
const isSuccess = await copyImage(img);
alert(`isSuccess: ${isSuccess}, 클립보드에 복사됐습니다. 브라우저 콘솔을 확인해주세요.`);
};
const handleReadText = async () => {
const isSuccess = await readText(text);
alert(`isSuccess: ${isSuccess}, 클립보드에 데이터를 가져왔습니다. 브라우저 콘솔을 확인해주세요.`);
};
const handleReadContents = async () => {
const isSuccess = await readContents(img);
alert(`isSuccess: ${isSuccess}, 클립보드에 데이터를 가져왔습니다. 브라우저 콘솔을 확인해주세요.`);
};
useEffect(() => {
console.log("copiedData: ", copiedData);
console.log("readData: ", readData);
}, [copiedData, readData])
return (
<div>
<div>
<input
type="text"
value={text}
onChange={(e) => setText(e.target.value)}
/>
<button onClick={handleCopyText}>텍스트 클립보드 복사</button>
</div>
<br />
<div>
<img src={img} alt="이미지" width={120} height={120} />
<button onClick={handleCopyImage}>이미지 클립보드 복사</button>
</div>
<br />
<div>
<button onClick={handleReadText}>클립보드 텍스트 읽기</button>
<button onClick={handleReadContents}>클립보드 컨텐츠 읽기</button>
</div>
</div>
);
};
Example
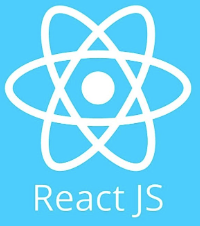